Exploring the Landscape of Android Databases
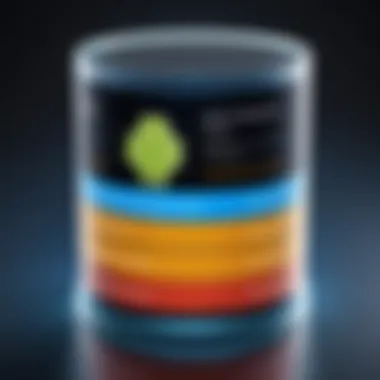
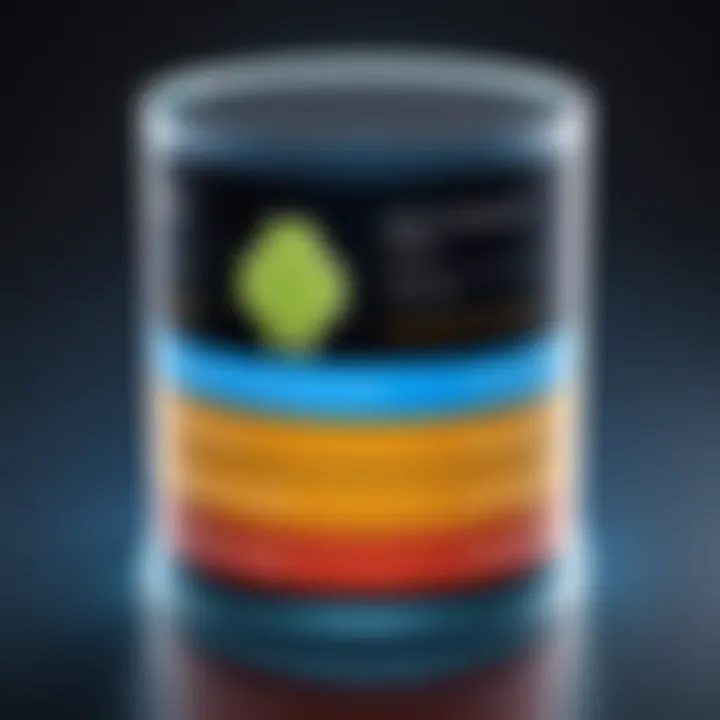
Intro
The world of Android databases is a critical aspect of mobile application development. For developers and businesses aiming to manage data effectively and efficiently, understanding the available database options is essential. This is especially true in a landscape characterized by constant innovation and change. In this exploration, we will delve into the nuances of database technologies utilized in Android applications, specifically focusing on SQLite and NoSQL databases such as Firebase Realtime Database and MongoDB.
As applications become more data-centric, the need for robust database management grows. Many developers face challenges when selecting the right database for their projects. This guide aims to clarify the options available and to outline best practices for implementation, optimization, and scalability.
Whether it's ensuring data persistence, enhancing performance, or managing database connections, the insights provided here will help IT professionals and business leaders make informed decisions related to their software procurement and deployment. As technology continues to evolve, being informed about database options can significantly impact the functionality and longevity of an application, ultimately leading to better user experiences and operational efficiencies.
Understanding Android Database Fundamentals
In any software application, databases play a crucial role in managing data efficiently and securely. For Android applications, a clear understanding of database fundamentals is essential for effective design and implementation. This section explore the main concepts and benefits of carefully structured databases within the Android framework.
Defining Android Databases
Android databases are structured collections of data that applications use to store, retrieve, and manage information. They differ from traditional file storage systems as they organize data in a way that allows for quick access and manipulation. The primary types of databases utilized in Android development are SQLite and NoSQL variants. SQLite is particularly notable for its lightweight nature and seamless integration with mobile applications, allowing developers to leverage an efficient, relational database management system directly on user devices.
The choice of an appropriate database has significant implications for the application's performance and user experience. Understanding various data management techniques ensures that applications can efficiently handle user data, even in the face of growing datasets or complex queries.
Key Components of Android Databases
Understanding the key components of Android databases allows developers to design their applications more effectively. These components include:
- Persistence Layer: This is where data is stored long-term, allowing retrieval even after the application is closed.
- Database Schema: The schema defines how the data is structured, including tables, columns, and relationships. A well-planned schema reduces redundancy and improves data integrity.
- Data Access Layer: This part manages how the application interacts with the database. It includes various methods for querying, inserting, updating, and deleting data, commonly referred to as CRUD operations.
- Indexing: Proper indexing of tables improves the speed of data retrieval. It is essential for maintaining performance, especially with large datasets.
- Transactions: These ensure that multiple operations are completed successfully or not executed at all, maintaining data integrity even in cases of failure.
Understanding these components not only enhances the database performance but also supports future scaling and maintenance efforts. Proper management of these elements can lead to a robust and efficient database architecture, ultimately benefiting the application's overall effectiveness.
"Effective database management is the backbone of a successful application, where performance meets user satisfaction."
The knowledge gained from understanding Android database fundamentals paves the way for exploring specific database types and implementation strategies in subsequent sections.
Types of Databases in Android
Understanding the various types of databases is crucial for effective data management in Android applications. As the demand for mobile applications increases, developers are presented with options for data storage that cater to different needs. Selecting the appropriate database type can significantly impact performance, scalability, and ease of development. This section will delve into two main categories: SQLite and NoSQL databases. Each offers unique features and benefits that can aid in the design and implementation of robust Android applications.
SQLite: The Default Database Solution
SQLite is the most widely used database for Android app development. Many developers choose SQLite due to its simplicity and lightweight structure. It operates as a local database, fitting seamlessly within the Android operating environment. SQLite is both efficient and easily accessible, making it an ideal choice for apps that require structured data storage but do not demand extensive server-side management.
One fundamental advantage of SQLite is its ACID compliance. This guarantees that all database transactions are processed reliably. Even in situations where a power failure occurs, SQLite ensures the integrity of the database. Additionally, SQLite enables developers to perform operations on data using the SQL language, which many are already familiar with. This familiarity speeds up the development process.
Here are some key features of SQLite in Android applications:
- Lightweight and fast: It minimizes overhead, which makes it suitable for mobile devices.
- Embedded: SQLite is embedded in the application, meaning no additional setup is necessary.
- Serverless: No configuration for a server environment is required, reducing complexity.
NoSQL Databases: An Emerging Alternative
NoSQL databases have surged in popularity as a flexible alternative to traditional relational databases like SQLite. They are particularly beneficial in scenarios where data structure may change frequently, or when applications require high scalability. NoSQL databases allow the storage of unstructured and semi-structured data, making them an attractive option for modern applications that handle diverse data types.
Common NoSQL databases used in Android development include Firebase Realtime Database and MongoDB. These databases excel in handling large volumes of data and complex data structures, such as JSON objects. This is particularly useful for applications focused on real-time data synchronization or those that need to fetch data from the cloud.
Key benefits of NoSQL databases include:
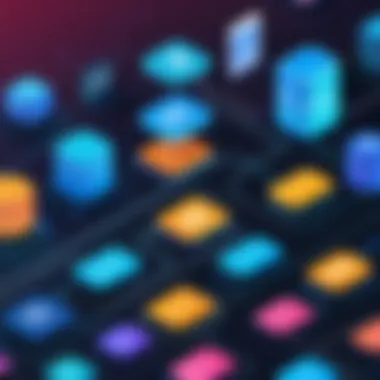
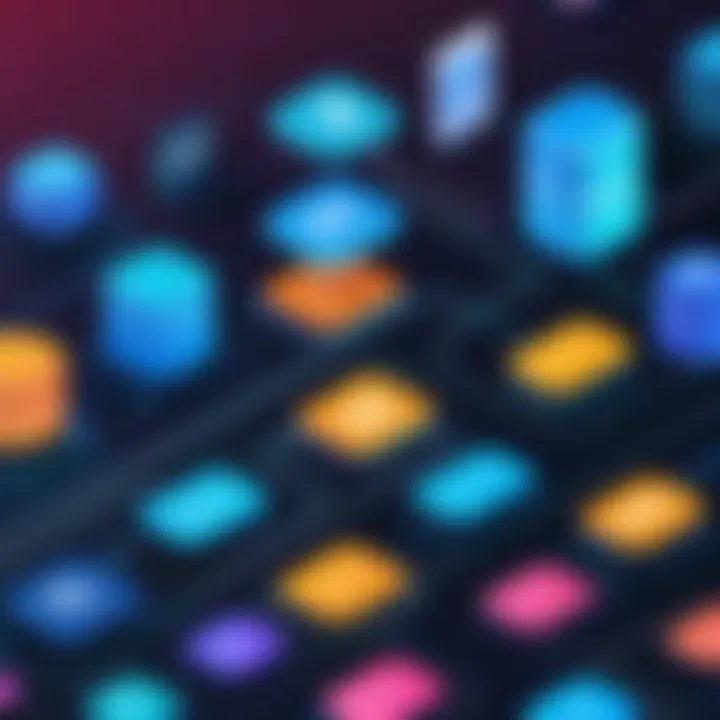
- Schema flexibility: Developers can easily adapt to changes in data requirements.
- Horizontal scalability: They can distribute data across many servers, providing high availability and fault tolerance.
- Faster data access: Data is often retrieved more quickly than it would be in traditional SQL databases due to its unstructured format.
Comparing SQLite and NoSQL
The choice between SQLite and NoSQL databases often depends on the specific requirements and constraints of the project. While SQLite provides an efficient method for managing structured data, NoSQL databases offer the flexibility needed for evolving data models.
SQLite might be more suitable when:
- Applications require a reliable, transactional database.
- There's a need for complex queries and structured data storage.
- You want to manage local device storage without relying on a server.
NoSQL databases might be better when:
- Applications handle large amounts of data that are semi-structured or unstructured.
- Real-time data access and flexibility are critical to application performance.
- Developers want a straightforward approach to scale databases horizontally.
Implementing SQLite in Android Apps
SQLite plays a crucial role in Android applications as the default database management system. Its lightweight nature and integration make it a popular choice for developers. Implementing SQLite ensures that apps can efficiently store and manage structured data, enhancing overall performance and user experience. This section will explore the practical steps involved in establishing SQLite databases within Android apps, focusing on their setup, the operations (commonly referred to as CRUD), and the considerations behind designing a robust database schema.
Setting Up SQLite Database
Setting up an SQLite database in an Android app is a fundamental task that involves creating a database instance and defining its structure. Android provides a straightforward and effective API for interacting with SQLite, which makes it accessible for developers.
The initial step is to derive a class from . This class assists in creating and managing your database. In this class, you need to override two methods: and .
After establishing your class, you can create an instance of it in your activities, which gives you access to your database. This process streamlines database setup and helps avoid complications regarding versioning.
CRUD Operations Explained
CRUD operations are essential for managing data within your SQLite database. They encompass Create, Read, Update, and Delete functions, each serving a unique purpose in interacting with the data.
Create
Create operations insert new records into the database. The method from the class is used here. This operation is essential because it allows applications to add data that users generate or enter.
The ability to create new records is crucial to user engagement and data utilization. A common characteristic of the Create operation is its straightforwardness, making it a popular choice among developers.
For instance, when a user registers on an app, a Create operation adds that user’s information to the database. This ensures data remains persistent. One advantage of using Create is the simplicity of implementing it, although it may increase the risk of duplicate entries if proper checks are not in place.
Read
Reading from the database is a critical operation that retrieves data. The method allows developers to access previously stored information. This operation is vital for displaying data, as it enables applications to utilize existing records efficiently.
The key characteristic of Read operations is their versatility. They can cater to various queries, from selecting all records to filtering based on specific conditions. A unique feature of Read is its ability to maintain user experience by providing instant access to data. However, improper queries could impact performance, making meticulous query optimization necessary.
Update
Updating stored data is a necessary function that keeps the database current and relevant. The method in SQLite allows existing records to be modified. An Update operation is particularly beneficial when data changes occur, such as a user editing their profile information.
Its key characteristic is responsiveness, allowing applications to adapt to user needs. A unique aspect of Update is its selectivity; developers can choose specific records to change, thereby minimizing resource use. However, careful validation is needed within this process to prevent unauthorised data alterations.
Delete
Delete operations remove records from the database, freeing up space and ensuring data accuracy. The method is implemented for this purpose. Keeping the database clean and up-to-date is essential. That's why Delete is beneficial in managing data.
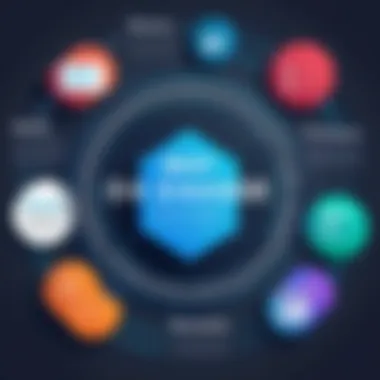
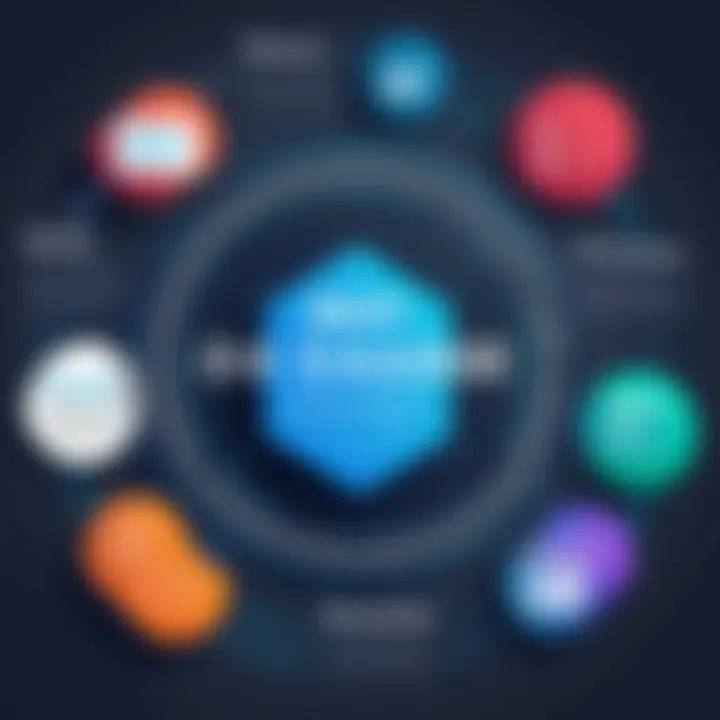
The key characteristic here is decisiveness; when data is no longer relevant or requested by a user, it needs removal. The unique feature of Delete is its irreversible nature, emphasizing careful consideration before executing the operation. If not handled properly, this operation can lead to data loss, making backups essential.
Database Schema Design Considerations
Effective database schema design is fundamental to successful application performance. Poorly designed schemas can lead to various issues, including slow performance and data redundancy. A robust schema considers relations among data, normalization to eliminate duplicates, and proper indexing for speedy access.
Aspects such as data types and constraints ensure integrity and consistency. Additionally, updating the schema to accommodate new requirements without disrupting existing systems is crucial. Proper design is a vital step to maintain the longevity and effectiveness of your application.
Using NoSQL Databases in Android Development
NoSQL databases are becoming vital in the Android development landscape. They serve specific use cases where traditional relational databases might struggle. In mobile applications, data can vary significantly in structure and volume. When developers work on applications that require flexibility and rapid scalability, NoSQL provides a robust solution. It is essential to explore how NoSQL databases fit into the Android ecosystem.
An Overview of NoSQL Options
NoSQL options come in various shapes. Popular choices include Firebase Firestore, MongoDB, and Couchbase. Each of these databases offers unique features:
- Firebase Firestore: Known for its real-time synchronization and easy integration with other Firebase services. It is a favorite for apps that need real-time updates.
- MongoDB: Features a document-based structure where data is stored in flexible, JSON-like documents. It allows for easy querying and indexing.
- Couchbase: Excels in performance and scalability, particularly for applications with large amounts of data across distributed systems.
Choosing the right NoSQL database depends on the specific requirements of the application. Key considerations should include data structure, access patterns, and expected scale.
Integrating NoSQL with Android Apps
Integrating NoSQL solutions into Android apps involves clear steps. The process typically includes SDK installation, configuration, and designing data models. Here is how one might approach it:
- Install the SDK: Most NoSQL databases provide an SDK for Android. Developers can add dependencies via Gradle.
- Configuration: This involves connecting the app to the database service. Proper authentication is critical here to ensure data security.
- Designing Data Models: In NoSQL, data modeling is less rigid than in relational systems. It is essential to understand how data will be stored and retrieved. For example, in Firestore, one can create collections and documents that relate to one another without the constraints of fixed schemas.
Code snippet for initializing Firebase Firestore in an Android project:
This snippet shows the simplicity of connecting to a NoSQL service. Future developers can quickly begin working with data once they establish this connection.
Advantages of NoSQL Databases
NoSQL databases bring numerous advantages to Android development, notably:
- Schema Flexibility: Developers can change the structure of the data without dealing with migrations typically necessary in relational databases.
- Scalability: These databases are designed to scale horizontally, allowing applications to handle increased loads with ease.
- Speed: They often provide better performance for read and write operations due to their less complex structures.
- Hierarchical Data Storage: Certain NoSQL databases allow natural storage of nested data without complex joins.
Best Practices for Android Database Management
Android database management requires a structured approach to ensure efficiency, security, and reliability. Implementing best practices is crucial for maintaining data integrity and optimizing application performance. These practices not only simplify development but also enhance user experience. Successful database management contributes directly to the overall health of an Android application.
Data Security Measures
Data security is paramount in Android development. Developers need to protect sensitive user information from potential breaches. Several measures can be taken to enhance security. Encryption is one of the most powerful tools. Encrypting databases ensures that even if data is compromised, it remains unreadable without the proper decryption keys. Android provides the library, which allows encryption of SQLite databases with minimal effort.
Additionally, securing data in transit is vital. Using HTTPS for communications prevents data interception and ensures secure interactions between the app and the server. Another important measure is implementing user authentication. Validating user credentials before granting access to sensitive data reinforces security. Implement access controls based on user roles to limit what data each user can interact with, reducing unnecessary exposure to information.
Performance Optimization Techniques
Optimizing database performance is essential for a smooth user experience. Poorly optimized databases can lead to slow application performance and frustrated users. One of the primary techniques involves indexing. Proper indexing can dramatically speed up data retrieval operations. However, be cautious; excessive indexing can slow down write operations, so balance is key.
Another technique involves optimizing queries. Use prepared statements instead of regular queries whenever possible. Prepared statements improve performance by allowing the database to cache query plans. Also, be mindful of the size of the database. Regularly prune or archive old data to keep the database lean. This prevents unnecessary slowdowns and enhances the overall performance of the application.
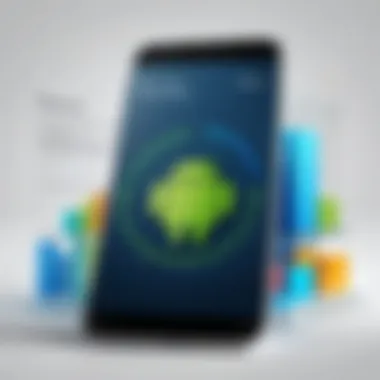
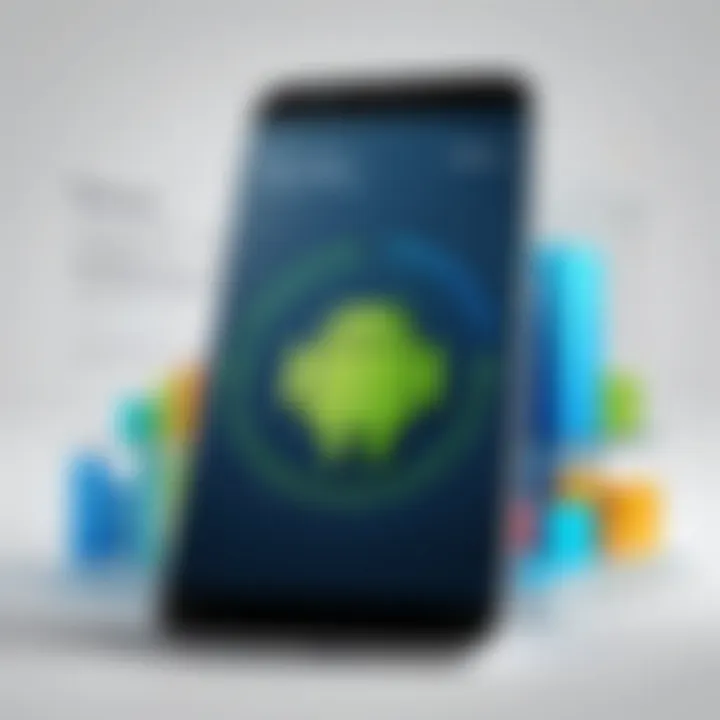
Regular Maintenance and Backup Strategies
Regular maintenance ensures the database operates at optimal efficiency. Routine checks should be conducted to identify any anomalies or issues within the database. This proactive approach allows for the quick resolution of potential issues before they affect the application.
Backups are another critical aspect of maintenance. Implement a reliable backup strategy to protect against data loss. Automated backups should be scheduled at regular intervals, ensuring data can be restored quickly in case of a failure. For enhanced security, consider storing backups in a secure location, separate from the main database. This minimizes risks associated with data loss due to hardware failures or security breaches.
"Proper database management is not just about storing data; it's about ensuring its integrity and security while optimizing performance."
Common Challenges in Android Database Usage
Understanding the common challenges in Android database usage is essential for developers and decision-makers alike. As applications demand more sophisticated storage mechanisms, challenges can arise that may hinder performance, data integrity, and overall user experience. Recognizing these issues can empower developers to implement more robust solutions and avoid pitfalls that can lead to costly setbacks.
Data Synchronization Issues
One of the significant challenges in Android database usage is data synchronization. As applications often rely on data from various sources, ensuring all data points reflect real-time information is crucial. When data is not synchronized, inconsistencies can arise, leading to confusing user experiences. This is particularly noticeable in applications that depend on cloud data or multiple devices.
- Impacted User Experience: Users may experience outdated information, creating frustration.
- Technical Complexities: Implementing efficient synchronization mechanisms can be complex, requiring careful planning and execution.
To improve synchronization, developers might employ background synchronization techniques. Using libraries such as WorkManager can help manage tasks efficiently, ensuring that data is consistently updated and in line with user expectations.
Performance Bottlenecks
Performance bottlenecks can severely degrade the speed and responsiveness of an application. These can be attributed to various factors, including inefficient database queries, excessive data loading, or resource-intensive processing tasks. It is essential to closely monitor key performance indicators to identify potential issues.
- Query Optimization: Queries that are not well-optimized will lead to longer load times. Developers can leverage tools to analyze and optimize SQL queries.
- Memory Management: Avoiding memory leaks and efficiently managing resources are critical for maintaining application performance.
Technical tools and strategies, such as indexing or using asynchronous tasks for data retrieval, provide a pathway to enhancing performance. This way, applications can handle more data while maintaining responsiveness.
Handling Corrupted Databases
Database corruption issues may arise from unexpected crashes, improper shutdowns, or even hardware malfunctions. Handling corrupted databases is vital for application stability and data integrity. A corrupted database can lead to unpredictable behavior, lost data, and negative user experiences.
- Detection and Recovery: Developers should implement strategies to automatically detect corruption and initiate recovery processes. Regular backups can also mitigate data loss.
- Graceful Degradation: Ensuring the application can degrade gracefully when corruption is detected is crucial. This means providing the user with a clear error message rather than allowing the app to crash.
Overall, understanding these common challenges equips developers to devise strategies that enhance the resilience and reliability of their applications. As the Android ecosystem continues to evolve, being aware of these potential obstacles can lead to better application design and improved user satisfaction as a result.
Future Trends in Android Databases
The landscape of Android databases is evolving rapidly. Understanding these future trends is crucial for professionals in the field. This section explores emerging dynamics, focusing on advancements that can aid developers and businesses in maximizing application performance and scalability.
Rise of Cloud Databases
Cloud databases are becoming more prevalent in Android development. They offer flexibility that is not always achievable with traditional database systems. For example, Google Cloud Firestore and Amazon DynamoDB provide robust, scalable architectures that support varying workloads and user demands.
Using cloud databases can reduce infrastructure costs. Instead of managing physical servers, companies can rely on powerful cloud providers. This means maintenance and operational tasks shift away from local teams, allowing them to focus on core functionalities.
Moreover, cloud databases usually provide automatic scaling. This means apps can handle increased loads without requiring manual adjustments. This is particularly vital for modern applications that see fluctuating usage patterns. With cloud-based solutions, data synchronization across devices becomes seamless as they manage data in real-time, allowing users to access the most current information regardless of their location.
Adoption of Machine Learning
Machine learning is increasingly influencing how databases are structured and utilized in Android development. Integrating machine learning within databases enhances their efficiency and predictive capabilities. For instance, the databases can leverage algorithms to analyze user behavior, improving data storage decisions.
By analyzing this data, applications can personalize user experiences. Developers can create smarter systems that offer recommendations or adjust functionalities based on user interactions. This level of customization was not feasible with more traditional approaches.
Additionally, machine learning can play a role in optimizing performance. Database management systems can use learning algorithms to predict heavy usage times and proactively allocate resources. This leads to maintaining responsiveness and preventing lags, ultimately resulting in better user satisfaction.
"The integration of machine learning within database systems is not just a trend; it is shaping the very foundation of how applications operate and manage data in real-time."
These advancements highlight the importance of staying informed about technological shifts. By being aware of these trends, IT professionals and businesses can make informed decisions about the tools and methods they adopt, ensuring that their applications remain relevant and competitive in a rapidly changing environment.